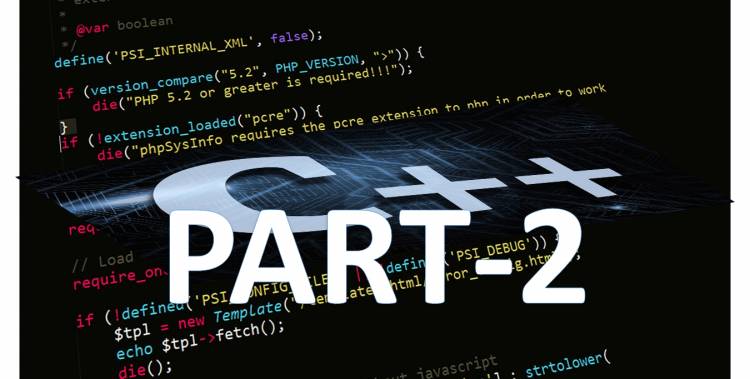
OOP CPP Programming language by Nadir Nadeem: Part-2
OOP CPP Programming Part-2
Lecture 5
Conditional statements:
- If- else (single condition)
- If- else if -else (multiple conditions)
- Switch-case (multiple conditions)
1:- if-else
Syntax:
If(condition){
Blok1
}
else{
block2(default block)
}
Example: #include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x=10; if(x>100){ cout<<"Ahmed"; } else{ cout<<"Danial"; }
return 0; } |
Output
2:- if else if- else
Syntax:
If(cond1){
Block1
}
else if(cond2){
block2
}
else if(cond3){
block3
}
else if(cond4){
block4
}
.
.
.
else{
default block
}
Example:#include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x=100; if(x>100){ cout<<x<<" is grater than 100"; } else if(x==100){ cout<<x<<" is equal to 100"; } else{ cout<<x<<" is less than 100"; }
return 0; } |
Output
Example:#include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x; cout<<"Enter a number:= ";cin>>x; if(x>100){ cout<<"value is grater than 100"; } else if(x==100){ cout<<"value is equal to 100"; } else{ cout<<"value is less than 100"; }
return 0; } |
Output
Student grade sheet:
90-100 A+
80-89 A
70-79 B
60-69 C
50-59 D
Fail
Example:#include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x; cout<<"Enter st test marks:= ";cin>>x; if(x>=90){ cout<<"Grade is A+"; } else if(x>=80){ cout<<"Grade is A"; } else if(x>=70){ cout<<"Grade is B"; } else if(x>=60){ cout<<"Grade is C"; } else if(x>=50){ cout<<"Grade is D"; } else{ cout<<"Student is Fail"; } return 0; } |
Output
Example:#include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x; cout<<"Enter st test marks:= ";cin>>x; if(x>=90){ cout<<"Grade is A+"<<endl; cout<<"Marvellous"<<endl; } else if(x>=80){ cout<<"Grade is A"<<endl; cout<<"Excellent"<<endl; } else if(x>=70){ cout<<"Grade is B"<<endl; cout<<"Very Good"<<endl; } else if(x>=60){ cout<<"Grade is C"<<endl; cout<<"Good"<<endl; } else if(x>=50){ cout<<"Grade is D"<<endl; cout<<"Average"<<endl; } else{ cout<<"Student is Fail"; } return 0; } |
Output
Example:#include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { char x; cout<<"Enter a, d, k or n:= ";cin>>x; if(x=='a'){ cout<<"Ahmad Bajwa"<<endl; } else if(x=='d'){ cout<<"Daniyal Chima"<<endl; } else if(x=='k'){ cout<<"Kazam Tatla"<<endl; } else if(x=='n'){ cout<<"Nadir Nadeem"<<endl; } else{ cout<<"Banday da puter ban tay likh a,d,k or n"; } return 0; } |
Output:
Lecture-2
3:- switch-case
- Related keywords
- break
- default
Syntax:
Switch(variable){
case cond1:{
block1
break;
}
case cond2:{
block2
break;
}
case cond3:{
block3
break;
}
.
.
.
}
default:{
default block
}
Example: #include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { char x; cout<<"Enter a, d, k or n:= ";cin>>x; switch(x){
case 'a': case 'A': { cout<<"Ahmed Ali"; break; } case 'd': case 'D': { cout<<"Daniyal Chima"; break; } default:{ cout<<"Not valid plz enter a,d,k or n"; } } return 0; } |
Output
Example: #include <iostream> #include <windows.h> using namespace std; int main(int argc, char** argv) { int x; cout<<"Enter a number:= ";cin>>x; switch(x){
case 10: { cout<<"TEN"; break; } case 20: { cout<<"Twenty"; break; } default:{ cout<<"Not valid plz a valid number"; } } return 0; } |
Assignments
Ass#9 My favorite color
Enter your name: Ali
Press r, g, b, or y: r
Your name is Ali and your favorite color is red
Ass#10 Student grade sheet
Enter st name:
Enter class : 9th
Enter test subject: Math
Enter total mark of test: 40
Obtain: 39
=====================================
Grade Result
===================================
St name:
Class
Subj
Tm
Obt
%age: 97%
Grade: A+
Remarks: Marvelous
Ass#11 simple calculation with menu:
Enter 1st value:= 9
Enter 2nd value:= 3
- Add
- Subtract
- Multiply
- Div
- Mod
- Exit
Enter your choice from 1 to 6: 1
exit() function
- exit from application
syntax:
- exit(0);
break keyword
- exit from block not from App
Syntax:
- break;
Ass#12 simple calculation with Symbols:
Enter 1st value:= 9
Enter 2nd value:= 3
Enter +, -, *, /, or %: and zero for exit:
Ass#13 country information
- Pakistan
- Chaina
- Iran
- Iraq
- Napal
- Exit
Enter your choice from 1 to 6: 1
===========================
Islamic republic of Pakistan
==========================
Capital:
Papulation:
Provinces:
National language:
Other languages:
National flag:
National Animal:
National game:
National bird:
Fonder of Pakistan:
Independence Day:
Ass#14 eligible for license (>=18 and <=80)
Enter your age: 18
You are eligible for license
Ass#15 eligible for Police force (>=18, and height 66 inch)
Enter your Name:=
Enter Your age := 18
Enter your height in inches: 58
Ass# 9,10,11,12,13,14,15 also with switch-case
Ass#16,17,18,19,20,21,22